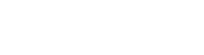 |
SCENE C++ API
2.1.0
|
3 #include <core_api/lsglobaldefinitions.h>
4 #include <core_api/lsinterface.h>
9 class LSString_Implementation;
30 CORE_API_EXPORT
LSString(
const wchar_t* str);
34 CORE_API_EXPORT
LSString(
const wchar_t& c);
82 CORE_API_EXPORT
const char*
toCharStr()
const;
89 CORE_API_EXPORT
const char*
toUtf8Str()
const;
95 CORE_API_EXPORT
const wchar_t*
toWcharStr()
const;
106 CORE_API_EXPORT
bool empty()
const;
112 CORE_API_EXPORT
int length()
const;
119 CORE_API_EXPORT
int find(
const LSString& str,
int pos = 0)
const;
126 CORE_API_EXPORT
int find(
const wchar_t* str,
int pos = 0)
const;
133 CORE_API_EXPORT
int find(
const wchar_t& c,
int pos = 0)
const;
140 CORE_API_EXPORT
int rfind(
const LSString& str,
int pos = -1)
const;
147 CORE_API_EXPORT
int rfind(
const wchar_t* str,
int pos = -1)
const;
154 CORE_API_EXPORT
int rfind(
const wchar_t& c,
int pos = -1)
const;
180 CORE_API_EXPORT
int compare(
const wchar_t* str)
const;
204 CORE_API_EXPORT
LSString&
replace(
const wchar_t* before,
const wchar_t* after);
210 CORE_API_EXPORT
LSString&
replace(
const wchar_t& before,
const wchar_t& after);
257 CORE_API_EXPORT
wchar_t operator[](
int pos)
const;
263 CORE_API_EXPORT
wchar_t&
at(
int pos);
269 CORE_API_EXPORT
wchar_t at(
int pos)
const;
280 CORE_API_EXPORT
void*
operator new(
size_t tSize);
285 CORE_API_EXPORT
void operator delete(
void* p);
309 CORE_API_EXPORT
LSString operator+(
const LSString& str1,
const wchar_t* str2);
316 CORE_API_EXPORT
LSString operator+(
const wchar_t* str1,
const LSString& str2);
337 CORE_API_EXPORT
bool operator== (
const LSString& str1,
const LSString& str2);
348 CORE_API_EXPORT
bool operator== (
const LSString& str1,
const wchar_t* str2);
354 CORE_API_EXPORT
bool operator== (
const wchar_t* str1,
const LSString& str2);
360 CORE_API_EXPORT
bool operator!= (
const LSString& str1,
const LSString& str2);
366 CORE_API_EXPORT
bool operator!= (
const LSString& str1,
const wchar_t* str2);
372 CORE_API_EXPORT
bool operator!= (
const wchar_t* str1,
const LSString& str2);
379 CORE_API_EXPORT
bool operator< (
const LSString& str1,
const LSString& str2);
386 CORE_API_EXPORT
bool operator< (
const LSString& str1,
const wchar_t* str2);
393 CORE_API_EXPORT
bool operator< (
const wchar_t* str1,
const LSString& str2);
400 CORE_API_EXPORT
bool operator<= (
const LSString& str1,
const LSString& str2);
407 CORE_API_EXPORT
bool operator<= (
const LSString& str1,
const wchar_t* str2);
414 CORE_API_EXPORT
bool operator<= (
const wchar_t* str1,
const LSString& str2);
421 CORE_API_EXPORT
bool operator> (
const LSString& str1,
const LSString& str2);
428 CORE_API_EXPORT
bool operator> (
const LSString& str1,
const wchar_t* str2);
435 CORE_API_EXPORT
bool operator> (
const wchar_t* str1,
const LSString& str2);
442 CORE_API_EXPORT
bool operator>= (
const LSString& str1,
const LSString& str2);
449 CORE_API_EXPORT
bool operator>= (
const LSString& str1,
const wchar_t* str2);
456 CORE_API_EXPORT
bool operator>= (
const wchar_t* str1,
const LSString& str2);